Notes on git
This page is managed by StJohn Piano.
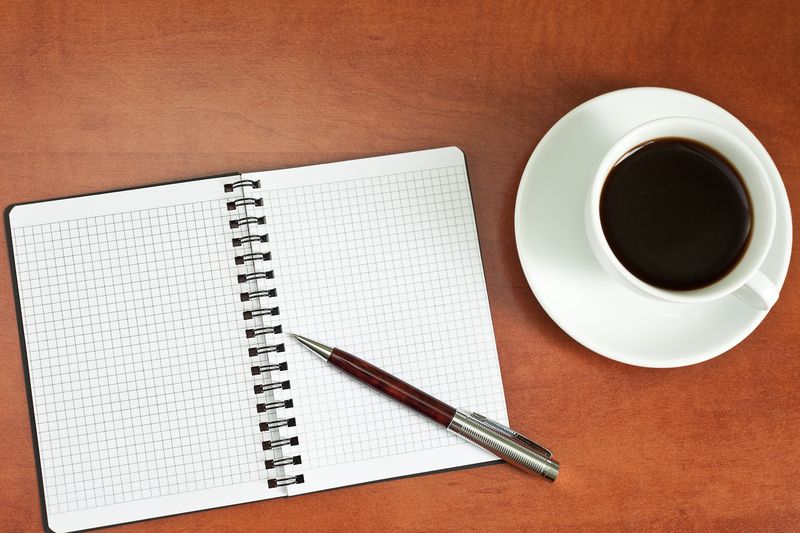
If you have any questions, comments, or suggestions - please contact StJohn Piano on Tela:
tela.app/id/stjohn_piano/7c51a6
Show colored history of all branches
git log --graph --oneline --all --decorate --abbrev-commit --date=format:'%Y-%m-%d %H:%M:%S' --format=format:'%C(bold blue)%h%C(reset) - %C(bold green)(%ad)%C(reset) %C(white)%s%C(reset) %C(bold white)- %an%C(reset)%C(bold yellow)%d%C(reset)'
To then use the shortcut git history
, add this function to .zshrc
:
git() {
if [[ $@ == "history" ]]; then
command git log --graph --oneline --all --decorate --abbrev-commit --date=format:'%Y-%m-%d %H:%M:%S' --format=format:'%C(bold blue)%h%C(reset) - %C(bold green)(%ad)%C(reset) %C(white)%s%C(reset) %C(bold white)- %an%C(reset)%C(bold yellow)%d%C(reset)'
else
command git "$@"
fi
}
.gitconfig file
% cat ~/.gitconfig
[user]
name = StJohn Piano
email = stjohn.piano@foo.com
[alias]
co = checkout
br = branch
ci = commit
st = status
log1 = log --oneline
who = blame
[init]
defaultBranch = main
[push]
autoSetupRemote = True
.zshrc file
alias g="git"
alias gs="git status"
alias ga="git add --patch"
alias gc="git commit"
alias gr="git reset HEAD"
Set git user globally and locally
# List git config
git config --list
# Set global git user
git config --global user.name "StJohn Piano"
git config --global user.email "stjohn.piano@foo.com"
# Set git user for local repo
git config user.name "StJohn Piano @ CompanyX"
git config user.email "stjohn.piano@companyx.com"
# Get git user from current config
# Note: Local config supersedes global config.
git config --get user.name
git config --get user.email
# See config for local repo
cd path/to/repo
cat .git/config
Create a new SSH key for your Github account
# Create a new SSH key
# Note: No passphrase is used.
SSH_EMAIL='stjohnpiano@foo.com'
SSH_FILENAME='id_rsa.github.username.computername'
SSH_FILEPATH=~/.ssh/$SSH_FILENAME
ssh-keygen -t rsa -b 4096 -C $SSH_EMAIL -f $SSH_FILEPATH -q -N ""
# Start SSH agent
eval "$(ssh-agent -s)"
# Add the SSH private key to the ssh-agent
ssh-add $SSH_FILEPATH
Now log on to Github and add your SSH key to your account. Here's the guide:
Github / Settings / SSH and GPG keys / New SSH key
Use this format for the key title:username@computername
If it's a workplace key, name it companyname_username@computername
instead.
Print the public key for pasting into the Key textarea:cat $SSH_FILEPATH.pub
Configure git repo to use SSH to connect to the remote
If you've cloned a repo from Github using HTTPS, then when you try to push, you'll be prompted for your Github username and password. It's more convenient to configure git to use your SSH key to connect, so that you avoid the prompt.
# See the name of the remote
git remote
# See the links to the remote
git remote --verbose
# See the current URL for the remote repo
# We assume that the remote's name is 'origin'.
git remote get-url origin
# Set the remote URL to use SSH.
# Format: git@github.com:username/reponame.git
# Note: Double-check that you've used a colon (not a slash) after "github.com".
git remote set-url origin git@github.com:sj-piano/python3-editable-installs-2.git
Configure git repo to use a different SSH key to connect to Github
Situation: You're at work, and your default git config uses the work Github SSH key, but you need to make some commits on a personal Github repo, using a personal Github key. However, you don't want to use your actual personal key (which should stay on your personal machine), so instead it's best to create a new personal key specifically for your workplace machine.
Create a new SSH key (following earlier guide in this article), using this format for the name:.ssh/id_rsa.github.companyname_username_personal.computername
Adjust your .ssh/config
file to contain these Host entries:
Host github.com
HostName github.com
IdentityFile ~/.ssh/id_ed25519.github.companyname_username.computername
Host github.com.personal
HostName github.com
IdentityFile ~/.ssh/id_rsa.github.companyname_username_personal.computername
You can test the connection by running:
ssh -T git@github.com
ssh -T git@github.com.personal
If you want to confirm that the correct key is used for each host, you can grep the debug output:
ssh -T git@github.com -v 2>&1 | grep 'identity file'
ssh -T git@github.com.personal -v 2>&1 | grep 'identity file'
Now, in the local git repo, change the remote URL to use the "personal" github Host entry in your SSH config.
# Set the remote URL to use the personal SSH host.
git remote set-url origin git@github.com.personal:sj-piano/python3-editable-installs-2.git
# Double-check
git remote get-url origin
Double-check that ssh-agent is running and that you've added the new key to it. Check the list of keys in ssh-agent by running:ssh-add -l
You should now be able to git push
to your personal repo with your new workplace personal key.
You can test by running:git push --dry-run
Sources
https://superuser.com/questions/1064197/how-to-switch-git-user-at-terminal
https://stackoverflow.com/questions/21160774/github-error-key-already-in-use
https://unix.stackexchange.com/questions/69314/automated-ssh-keygen-without-passphrase-how